-
Create Entity Object
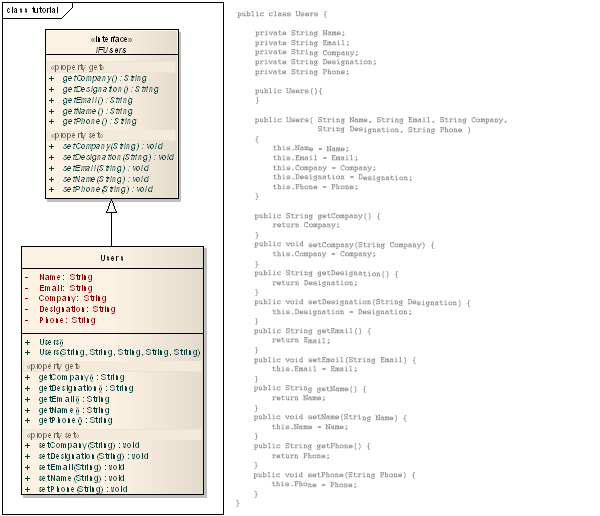
-
Create Simple Table by passing Entity as parameter in the Constructor
SimpleTable simpleTable = new SimpleTable(new Users());
contentPane.add(simpleTable, "Center");
Add Dummy Users
simpleTable.AddRow(new Users("Anna", "anna+christalign.com", "Christalign Innovative Solutions Pvt. Ltd.", "CEO", "+91 471 2384497"));
simpleTable.AddRow(new Users("Ruben", "ruben_gerad+christalign.com", "Christalign Innovative Solutions Pvt. Ltd.", "CTO", "+91 471 2384497"));
simpleTable.AddRow(new Users("Rubeela", "rubeela+christalign.com", "Christalign Innovative Solutions Pvt. Ltd.", "HR", "+91 471 2384497"));
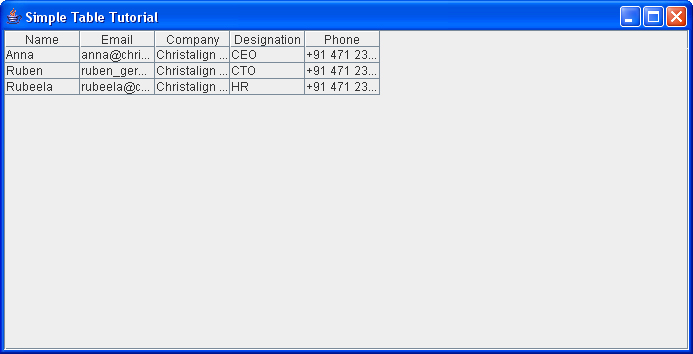
-
Now Resize columns and add a background image
private ImageIcon imgBackground = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/background.jpg"));
Set Table Properties
simpleTable.setTableResizeMode(JTable.AUTO_RESIZE_LAST_COLUMN);
simpleTable.setBackground(imgBackground);
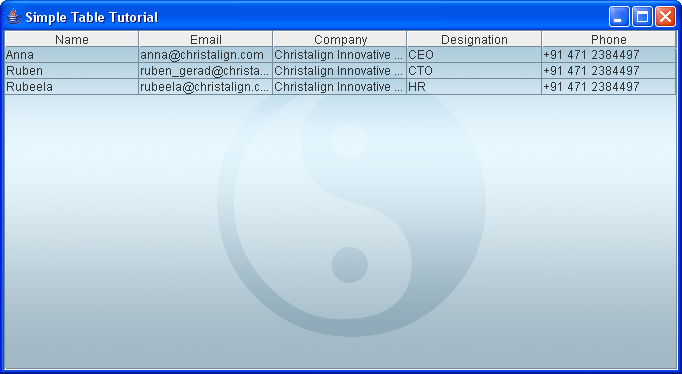
-
Add Header Image
private ImageIcon imgBackground = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/background.jpg"));
private ImageIcon imgDesignation = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/designation.jpg"));
Set Table Properties
simpleTable.setTableResizeMode(JTable.AUTO_RESIZE_LAST_COLUMN);
simpleTable.setBackground(imgBackground);
simpleTable.setColumnHeader("Designation", 72, imgDesignation );
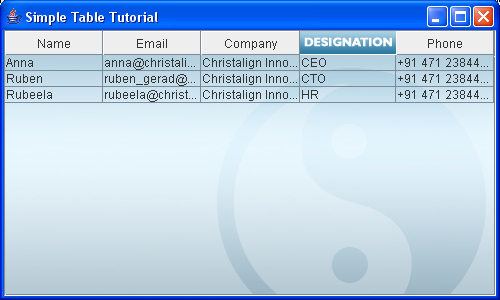
Fails to resize header image and render column correctly
Designation field has no background filling so there is space between designation and phone columns.

-
Use Header with background image
private ImageIcon imgBackground = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/background.jpg"));
private ImageIcon imgDesignation = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/designation.jpg"));
private ImageIcon imgName = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/name.jpg"));
private ImageIcon imgEmail = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/email.jpg"));
private ImageIcon imgDesignation = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/designation.jpg"));
private ImageIcon imgCompany = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/company.jpg"));
private ImageIcon imgPhone = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/phone.jpg"));
private ImageIcon imgHDBG = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/hdbg.jpg"));
Set Table Properties
simpleTable.setTableResizeMode(JTable.AUTO_RESIZE_LAST_COLUMN);
simpleTable.setBackground(imgBackground);
simpleTable.setColumnHeader("Name", 100, imgName, false, imgHDBG, imgHDBG, imgHDBG );
simpleTable.setColumnHeader("Email", 170, imgEmail, false, imgHDBG, imgHDBG, imgHDBG );
simpleTable.setColumnHeader("Designation", 100, imgDesignation, false, imgHDBG, imgHDBG, imgHDBG );
simpleTable.setColumnHeader("Company", 250, imgCompany, false, imgHDBG, imgHDBG, imgHDBG );
simpleTable.setColumnHeader("Phone", 100, imgPhone, false, imgHDBG, imgHDBG, imgHDBG );
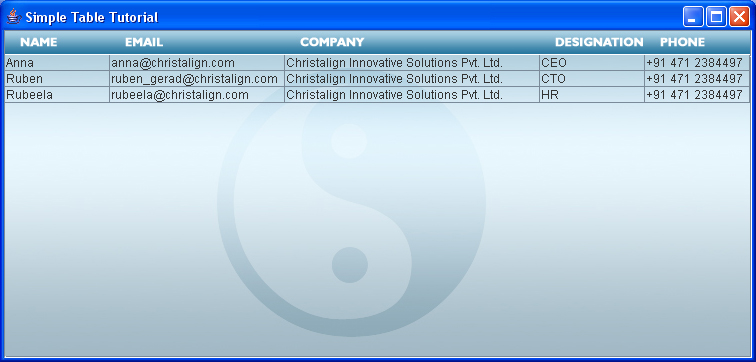
-
Use Header with background image, left and right border images.
private ImageIcon imgBackground = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/background.jpg"));
private ImageIcon imgName = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/name.jpg"));
private ImageIcon imgEmail = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/email.jpg"));
private ImageIcon imgDesignation = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/designation.jpg"));
private ImageIcon imgCompany = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/company.jpg"));
private ImageIcon imgPhone = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/phone.jpg"));
private ImageIcon imgHDBG = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/hdbg.jpg"));
private ImageIcon imgHDLeftBG = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/leftbg.jpg"));
private ImageIcon imgHDRightBG = new ImageIcon(com.christalign.emc.tutorial.SimpleTableTutorial.class.getResource("resources/rightbg.jpg"));
Set Table Properties
//simpleTable.setTableResizeMode(JTable.AUTO_RESIZE_LAST_COLUMN); //Disable to use scroll bar.
simpleTable.setBackground(imgBackground);
simpleTable.setColumnHeader("Name", 100, imgName, false, imgHDBG, imgHDLeftBG, imgHDRightBG );
simpleTable.setColumnHeader("Email", 170, imgEmail, false, imgHDBG, imgHDLeftBG, imgHDRightBG );
simpleTable.setColumnHeader("Designation", 100, imgDesignation, false, imgHDBG, imgHDLeftBG, imgHDRightBG );
simpleTable.setColumnHeader("Company", 250, imgCompany, false, imgHDBG, imgHDLeftBG, imgHDRightBG );
simpleTable.setColumnHeader("Phone", 100, imgPhone, false, imgHDBG, imgHDLeftBG, imgHDRightBG );
Additional Properties
CENTER IMAGE HEADERS
simpleTable.setColumnHeader("Name", 100, imgName, true, imgHDBG, imgHDLeftBG, imgHDRightBG );
simpleTable.setColumnHeader("Email", 175, imgEmail, true, imgHDBG, imgHDLeftBG, imgHDRightBG );
simpleTable.setColumnHeader("Company", 250, imgCompany, true, imgHDBG, imgHDLeftBG, imgHDRightBG );
simpleTable.setColumnHeader("Phone", 100, imgPhone, true , imgHDBG, imgHDLeftBG, imgHDRightBG );
INCREASE ROW HEIGHT
simpleTable.setRowHeight(35);
CHANGE GRID COLOR
simpleTable.setGridColor(Color.LIGHT_GRAY);
ENABLE MOUSE SCROLLING
simpleTable.setWheelScrollingEnabled(true);
SINGLE SELECTION MODE
simpleTable.setSelectionMode(ListSelectionModel.SINGLE_SELECTION);
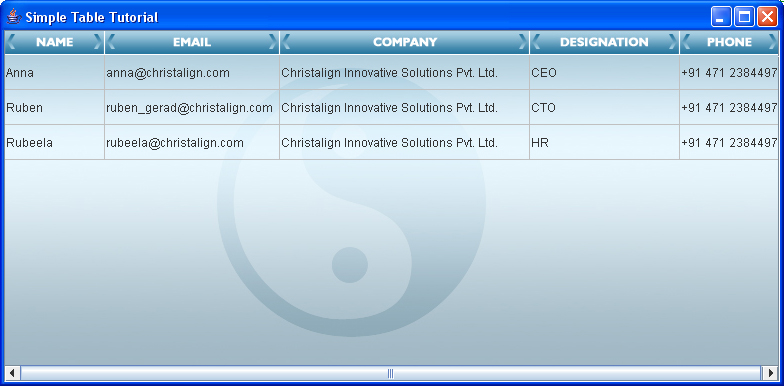
-
Adding Listeners
simpleTable.getSelectionModel().addListSelectionListener(new ListSelectionListener()
{
public void valueChanged(ListSelectionEvent e) {
if(e.getValueIsAdjusting())
return;
ListSelectionModel rowSM = (ListSelectionModel)e.getSource();
simpleTable.setCurrentRow(rowSM.getMinSelectionIndex());
}
});
- CRUD Operations
ADD Operation
simpleTable.AddRow(new Users("USER", "USER+XYZ.COM", "XYZ CORP","DGM", "33-070777"));
UPDATE Operation
simpleTable.UpdateRow(0, new Users("Anna", "anna+christalign.com", "Christalign Innovative Solutions Pvt. Ltd.", "CEO", "+91 9747007078"));
DELETE Operation
simpleTable.DeleteRow(2);
LIST Operation
for(int i=0; i<simpleTable.getRowCount(); i++){
Users user = (Users) simpleTable.getRow(i);
System.out.println(user.getName());
System.out.println(user.getEmail());
System.out.println(user.getCompany());
System.out.println(user.getDesignation());
System.out.println(user.getPhone());
}